1. What is a serverless application?
A combination of lambda functions and other services like S3, API Gateway etc. that work together to perform tasks.
Use cases:
- Backend APIs: that service web and mobile applications
- Static web applications
- Parallel compute
- Email processing
- Cron Jobs
2. Setup local development environment
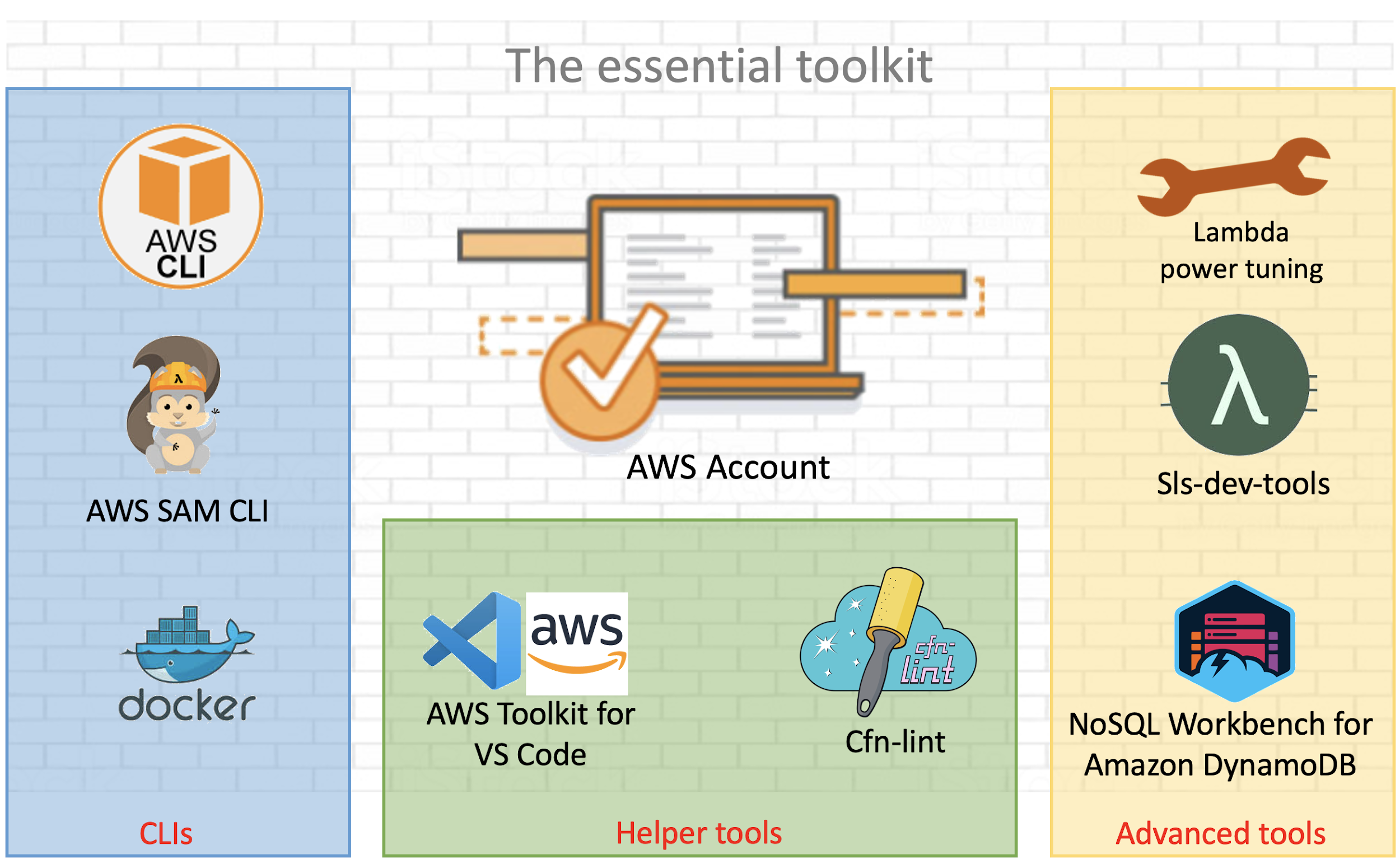
- Create IAM user: The IAM user that you use with AWS SAM must have sufficient permissions to make necessary AWS service calls and manage AWS resources
- Configure AWS credentials: The AWS SAM CLI requires you to set AWS credentials so that it can make calls to AWS services on your behalf. We can configure in different ways
- Awscli : install awscli, run aws configure and provide access key id and secret access key
- Environment variables: set environment variables AWS_ACCESS_KEY_ID and AWS_SECRET_ACCESS_KEY
- Credentials file: set credentials in the AWS credentials file on your local system.
- path for linux and macOS : ~/.aws/credentials
- path for windows os : C:\Users\USERNAME\.aws\credentials
- Docker (optional ) : AWS SAM provides a local environment that’s similar to AWS Lambda to use as a Docker container. You can use this container to build, test, and debug your serverless applications.
- Python: Language for serverless functions.
- AWS SAM CLI : This makes it easy to create and manage serverless applications
3. Create Sample project
#Step 1 - Download a sample application
sam init
#Step 2 - Build your application
cd sam-app
sam build
#Step 3 - Deploy your application
sam deploy --guided
4. SAM Commands
- sam init
- Initializes a serverless application with an AWS SAM template. The template provides a folder structure. This application includes everything that you need to get started and to eventually extend it into a production-scale application
- This is the first step to initialize the project.
- sam validate
- Verifies whether an AWS SAM template file is valid.
- sam build
- The sam build command processes your AWS SAM template file, application code, and any applicable language-specific files and dependencies.
- The build command creates the build directory in .aws-sam/build and installs the Python dependencies and the Lambda function ready for local testing or deployment
- sam package
- Zips up and upload function packages to s3.
- The command will produce another cloudformation template . The difference is the codeUri property of lambda function points to remote s3 bucket instead of local directory
- sam deploy
- Creates a running instance of the application, i.e. cloud formation stack based on the template.
- sam logs
- Fetches logs that are generated by your Lambda function.
- sam invoke
- Invokes a local AWS Lambda function once and quits after invocation completes
- sam local start-api
- Allows you to run your serverless application locally for quick development and testing. When you run this command in a directory that contains your serverless functions and your AWS SAM template, it creates a local HTTP server that hosts all of your functions.
- It starts a Docker container locally to invoke the function
- It can take a while for the Docker image to load. After it’s loaded, you can use curl to send a request to your application that’s running on your local host:
- sam local start-lambda
- Enables you to programmatically invoke your Lambda function locally by using the AWS CLI or SDKs.
- This command starts a local endpoint that emulates AWS Lambda. You can run your automated tests against this local Lambda endpoint.You can run your automated tests against this local Lambda endpoint.
# Invoking function with event file
$ sam local invoke "Ratings" -e event.json
# Invoking function with event via stdin
$ echo '{"message": "Hey, are you there?" }' | sam local invoke --event - "Ratings"
5. SAM Template sections
AWS SAM templates can include several major sections. Only the Transform and Resources sections are required. You can include template sections in any order.
- Headers
- AWSTemplateFormatVersion
- AWSTemplateFormatVersion: ‘2010-09-09’
- The AWSTemplateFormatVersion section (optional) identifies the capabilities of the template
- Transform
- Transform: ‘AWS::Serverless-2016-10-31’
- This declaration identifies an AWS CloudFormation template file as an AWS SAM template file
- Description
- The Description section (optional) enables you to include comments about your template.
- AWSTemplateFormatVersion
- Globals
- Sometimes resources that you declare in an AWS SAM template have common configurations. For example, the runtime of lambda functions.
- Instead of duplicating this information in every resource, you can declare them once in the Globals section and let your resources inherit them.
- Resources can override the properties that you declare in the Globals section
- Parameters
- Parameters enable you to input custom values to your template each time you create or update a stack. Example RAM size.
- You can reference parameters from the Resources and Outputs sections of the same template.
- Values that are passed in using the –parameter-overrides parameter of the sam deploy command and entries in the configuration file take precedence over entries in the AWS SAM template file
- Mappings
- The Mappings section consists of the key name Mappings. The keys in mappings must be literal strings. The values can be String or List types
- You can use the Fn::FindInMap function to return a named value based on a specified key.
- Conditionals
- Conditions control whether certain resources are created or whether certain resource properties are assigned a value during stack creation or update.
- Resources
- In AWS SAM templates the Resources section can contain a combination of AWS CloudFormation resources and AWS SAM resources. Example lambda function, API, DynamoDb table etc.
- Types
- AWS::Serverless::Api
- AWS::Serverless::Application
- AWS::Serverless::Function
- AWS::Serverless::HttpApi
- AWS::Serverless::LayerVersion
- AWS::Serverless::SimpleTable
- AWS::Serverless::StateMachine
- Outputs
- The values that are returned whenever you view your stack’s properties
6. Intrinsic functions
Intrinsic functions are built-in functions that enable you to assign values to properties that are only available at runtime.
Examples
- Ref ->Returns the value of the specified parameter or resource.
- Fn::FindInMap -> Returns the value corresponding to keys in a two-level map that is declared in the Mappings section.
- Fn::GetAtt -> Returns the value of an attribute from a resource in the template.
- Fn::GetAZs -> Returns an array that lists Availability Zones for a specified region in alphabetical order.
6. Safe Deployments
It is a good practice to expose your new code to a small percentage of production traffic, run tests, watch for alarms and dial up traffic as you gain more confidence. The goal is to minimize production impact as much as possible.
You can enable automated traffic shifting Lambda deployments by adding the following lines to your AWS::Serverless::Function resource property or in the Globals section.
AutoPublishAlias: live
DeploymentPreference:
Type: Linear10PercentEvery10Minutes
- AutoPublishAlias
- Create an Alias with
- Create & publish a Lambda version with the latest code & configuration derived from the CodeUri property. Optionally it is possible to specify property AutoPublishCodeSha256 that will override the hash computed for Lambda CodeUri property.
- Point the Alias to the latest published version
- Point all event sources to the Alias & not to the function
- When the CodeUri property of AWS::Serverless::Function changes, SAM will automatically publish a new version & point the alias to the new version
- Create an Alias with
- Deployment Preference Type
- CanaryXPercentYMinutes
- Traffic is shifted in two increments
- X percent of traffic will be routed to the new version for Y minutes. After Y minutes, 100 percent of traffic will be sent to the new version. Some people call this as Blue/Green deployment.
- Ex: Canary10Percent15Minutes will send 10 percent traffic to new version and 15 minutes later complete deployment by sending all traffic to new version
- LinearXPercentYMinutes
- Traffic to the new version will linearly increase in steps of X percentage every Y minutes.
- Ex: Linear10PercentEvery10Minutes will add 10 percentage of traffic every 10 minute to complete in 100 minutes.
- All-at-once
- This is an instant shifting of 100% of traffic to the new version. This is useful if you want to run pre/post hooks but don’t want a gradual deployment.
- CanaryXPercentYMinutes
7. Nested Applications
Nested applications or stacks are stacks that contain one or more other applications.
AWS::Serverless::Application embeds a serverless application from s3 bucket or from local file system. Nested applications are deployed as nested stacks, which can contain multiple other resources, including other AWS::Serverless::Application resources.
Resources:
MyApplication:
Type: AWS::Serverless::Application
Properties:
Location:
ApplicationId: 'arn:aws:serverlessrepo:us-east-1:012345678901:applications/my-application'
SemanticVersion: 1.0.0
Parameters:
StringParameter: parameter-value
IntegerParameter: 2
MyOtherApplication:
Type: AWS::Serverless::Application
Properties:
Location: https://s3.amazonaws.com/demo-bucket/template.yaml
Outputs:
MyNestedApplicationOutput:
Value: !GetAtt MyApplication.Outputs.ApplicationOutputName
Description: Example nested application output
8. CI / CD ( in Jenkins )
CICD pipeline for deploying serverless applications. This section covers only for Jenkins.
- AWS SAM Plugin. Link https://plugins.jenkins.io/aws-sam/
- Pipeline as code
stage('Prepare Build Environment') {
sh """
python3 -m venv venv
. venv/bin/activate
pip install aws-sam-cli
"""
env.PATH = "${env.WORKSPACE}/venv/bin:${env.WORKSPACE}/bin:${env.PATH}"
}
stage('Build Lambda Job') {
sh """
sam package \
--template-file template.yaml \
--output-template-file package.yml \
--s3-bucket ${params.project}-lambda \
--s3-prefix ${key}
"""
}
stage('Deploy Lambda Job') {
sh """
sam deploy \
--template-file package.yml \
--stack-name ${key} \
--capabilities CAPABILITY_IAM \
--no-fail-on-empty-changeset
"""
}
Reference: https://austincloud.guru/2020/06/18/deploying-sam-with-jenkins/